I'm busy writing a simple Egg Timer Application in my spare time for
my Mobile Phone (I keep forgetting to switch the damn water off when
watering the plants / filling the pool!)
One of the things I needed to do was to play some sound when the time
ran out. The sound file also needed to be embedded in the application
so as to avoid dependencies (which tend to cause trouble).
I found this article from M$.
I had specific problem with this:
Sound sound = new Sound(Assembly.GetExecutingAssembly().GetManifestResourceStream("SoundSample.chimes.wav"));
sound.Play();
I spent quite a bit of time trying to get the sound loaded, but to no avail.
Here's the work-around:
1) Add a sound file as a resource:
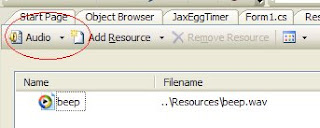
- Open the Resources.resx file of the project, and add an Audio Resource
- Select "Audio" type, and click "Add Resource"
- Browse to the sound file you would like to use (*.wav)
2) Copy the Sound class from the above M$ article, but change the constructor that takes a Stream, and change it to a byte[] parameter. Like so:
public Sound(byte[] soundBytes)
{
m_soundBytes = soundBytes;
}
3) Then instead of using GetManifestResourceStream(), pass sound bytes into the constructor if the Sound class:
eg:
Sound sound = new Sound(JaxEggTimer.Properties.Resources.beep);
sound.Play();
If anyone has managed to get the GetManifestResourceStream thing to work, please let me know how. (Leave a comment for others to see)
3 comments:
Hi
>>If anyone has managed to get the GetManifestResourceStream thing to work<<
You won't get it to work for an embedded resource, only an external file. Your method is good for the embedded resource option. You can declare an alternative constructor for the sound class as:
public Sound(byte[] soundBytes)
{
m_soundBytes = soundBytes;
}
which can exist as well as the MS stream constructor:
public Sound(Stream stream)
using System.Media;
SoundPlayer auf = new SoundPlayer(Thumbs2008.Properties.Resources.auf);
auf.Play();
tested on c# express 2008
Thumbs2008=your namespacename
you can download the result on http://oktay.com/thumbs2008/Thumbs2008.zip
under settings you will find the sound on settings
;-)
I know this is kind of old, but I've found that Assembly.GetExecutingAssembly() does not work as reliably as this.GetType().Assembly.
My code was:
Assembly myAssembly = Assembly.GetExecutingAssembly();
SoundPlayer sound = new Sound();
sound.Stream = myAssembly.GetResourceManifestStream("Namespace.warning.wav");
sound.Play();
Changed line 1 to:
Assembly myAssembly = this.GetType().Assembly;
Post a Comment